python之⾯向对象基础
⼀. 理解⾯向对象
⼆. 类和对象
2.1 理解类和对象
2.1.1 类
- 特征即是属性
- ⾏为即是⽅法
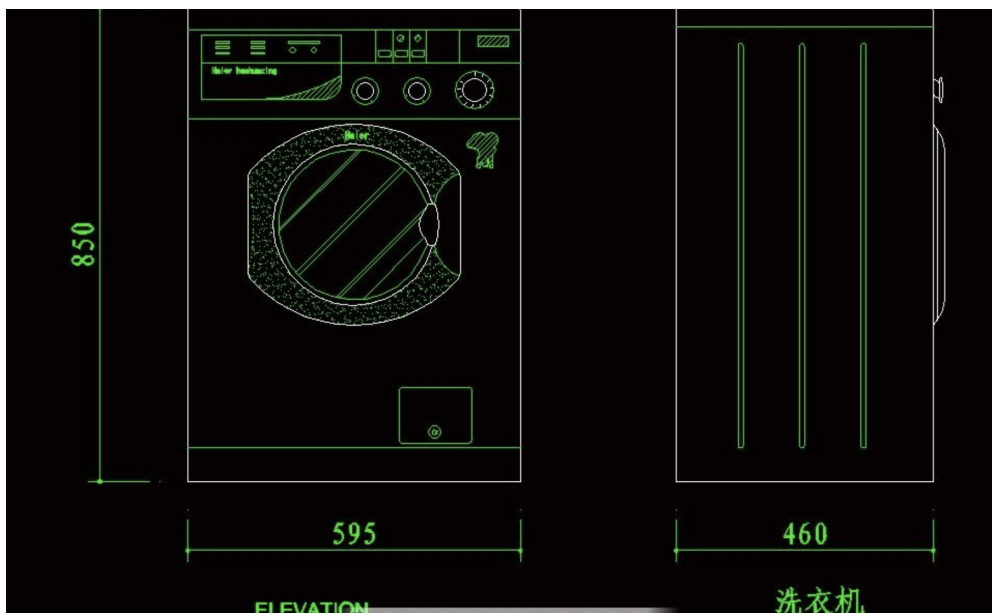
2.1.2 对象
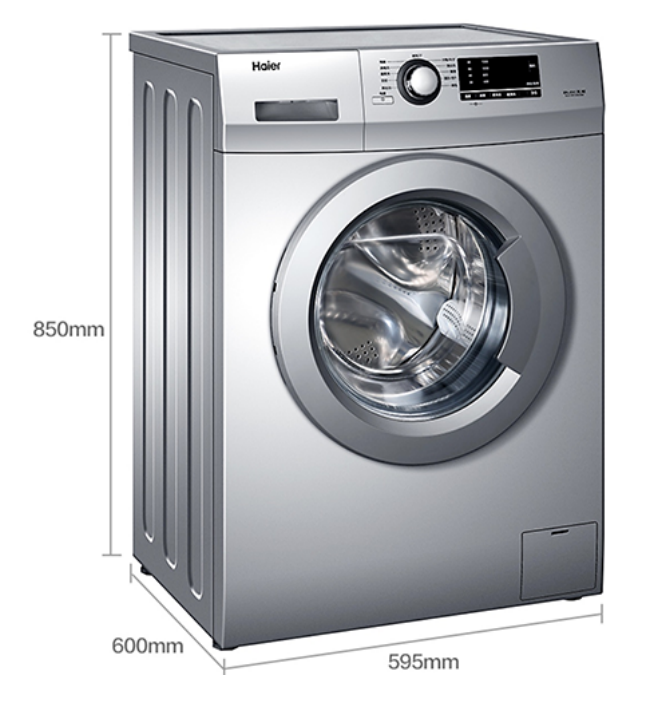
2.2 ⾯向对象实现⽅法
2.2.1 定义类
class 类名():
代码
......
class Washer():
def wash(self):
print('我会洗⾐服')
class 类名:
代码
......
2.2.2 创建对象
对象名 = 类名()
# 创建对象
haier1 = Washer()
# <__main__.Washer object at 0x0000018B7B224240>
print(haier1)
# haier对象调⽤实例⽅法
haier1.wash()
2.2.3 self
# 1. 定义类
class Washer():
def wash(self):
print('我会洗⾐服')
# <__main__.Washer object at 0x0000024BA2B34240>
print(self)
# 2. 创建对象
haier1 = Washer()
# <__main__.Washer object at 0x0000018B7B224240>
print(haier1)
# haier1对象调⽤实例⽅法
haier1.wash()
haier2 = Washer()
# <__main__.Washer object at 0x0000022005857EF0>
print(haier2)
三. 添加和获取对象属性
3.1 类外⾯添加对象属性
对象名.属性名 = 值
haier1.width = 500
haier1.height = 800
3.2 类外⾯获取对象属性
对象名.属性名
print(f'haier1洗⾐机的宽度是{haier1.width}')
print(f'haier1洗⾐机的⾼度是{haier1.height}')
3.3 类⾥⾯获取对象属性
self.属性名
# 定义类
class Washer():
def print_info(self):
# 类⾥⾯获取实例属性
print(f'haier1洗⾐机的宽度是{self.width}')
print(f'haier1洗⾐机的⾼度是{self.height}')
# 创建对象
haier1 = Washer()
# 添加实例属性
haier1.width = 500
haier1.height = 800
haier1.print_info()
四. 魔法⽅法
4.1 __init__()
4.1.1 体验__init__()
class Washer():
# 定义初始化功能的函数
def __init__(self):
# 添加实例属性
self.width = 500
self.height = 800
def print_info(self):
# 类⾥⾯调⽤实例属性
print(f'洗⾐机的宽度是{self.width}, ⾼度是{self.height}')
haier1 = Washer()
haier1.print_info()
4.1.2 带参数的__init__()
class Washer():
def __init__(self, width, height):
self.width = width
self.height = height
def print_info(self):
print(f'洗⾐机的宽度是{self.width}')
print(f'洗⾐机的⾼度是{self.height}')
haier1 = Washer(10, 20)
haier1.print_info()
haier2 = Washer(30, 40)
haier2.print_info()
4.2 __str__()
class Washer():
def __init__(self, width, height):
self.width = width
self.height = height
def __str__(self):
return '这是海尔洗⾐机的说明书'
haier1 = Washer(10, 20)
# 这是海尔洗⾐机的说明书
print(haier1)
4.3 __del__()
class Washer():
def __init__(self, width, height):
self.width = width
self.height = height
def __del__(self):
print(f'{self}对象已经被删除')
haier1 = Washer(10, 20)
# <__main__.Washer object at 0x0000026118223278>对象已经被删除
del haier1
五. 综合应⽤
5.1 烤地⽠
5.1.1 需求
- 0-3分钟:⽣的
- 3-5分钟:半⽣不熟
- 5-8分钟:熟的
- 超过8分钟:烤糊了
- ⽤户可以按⾃⼰的意愿添加调料
5.1.2 步骤分析
5.1.2.1 定义类
- 被烤的时间
- 地⽠的状态
- 添加的调料
- 被烤
-
⽤户根据意愿设定每次烤地⽠的时间
-
判断地⽠被烤的总时间是在哪个区间,修改地⽠状态
- 添加调料
-
⽤户根据意愿设定添加的调料
-
将⽤户添加的调料存储
5.1.2.2 创建对象,调⽤相关实例⽅法
5.1.3 代码实现
5.1.3.1 定义类
- 定义地⽠初始化属性,后期根据程序推进更新实例属性
class SweetPotato():
def __init__(self):
# 被烤的时间
self.cook_time = 0
# 地⽠的状态
self.cook_static = '⽣的'
# 调料列表
self.condiments = []
5.1.3.2 定义烤地⽠⽅法
class SweetPotato():
......
def cook(self, time):
"""烤地⽠的⽅法"""
self.cook_time += time
if 0 <= self.cook_time < 3:
self.cook_static = '⽣的'
elif 3 <= self.cook_time < 5:
self.cook_static = '半⽣不熟'
elif 5 <= self.cook_time < 8:
self.cook_static = '熟了'
elif self.cook_time >= 8:
self.cook_static = '烤糊了'
5.1.3.3 书写str魔法⽅法,⽤于输出对象状态
class SweetPotato():
......
def __str__(self):
return f'这个地⽠烤了{self.cook_time}分钟, 状态是{self.cook_static}'
5.1.3.4 创建对象,测试实例属性和实例⽅法
digua1 = SweetPotato()
print(digua1)
digua1.cook(2)
print(digua1)
5.1.3.5 定义添加调料⽅法,并调⽤该实例⽅法
class SweetPotato():
......
def add_condiments(self, condiment):
"""添加调料"""
self.condiments.append(condiment)
def __str__(self):
return f'这个地⽠烤了{self.cook_time}分钟, 状态是{self.cook_static}, 添加的调料有{self.condiments}'
digua1 = SweetPotato()
print(digua1)
digua1.cook(2)
digua1.add_condiments('酱油')
print(digua1)
digua1.cook(2)
digua1.add_condiments('辣椒⾯⼉')
print(digua1)
digua1.cook(2)
print(digua1)
digua1.cook(2)
print(digua1)
5.1.4 代码总览
# 定义类
class SweetPotato():
def __init__(self):
# 被烤的时间
self.cook_time = 0
# 地⽠的状态
self.cook_static = '⽣的'
# 调料列表
self.condiments = []
def cook(self, time):
"""烤地⽠的⽅法"""
self.cook_time += time
if 0 <= self.cook_time < 3:
self.cook_static = '⽣的'
elif 3 <= self.cook_time < 5:
self.cook_static = '半⽣不熟'
elif 5 <= self.cook_time < 8:
self.cook_static = '熟了'
elif self.cook_time >= 8:
self.cook_static = '烤糊了'
def add_condiments(self, condiment):
"""添加调料"""
self.condiments.append(condiment)
def __str__(self):
return f'这个地⽠烤了{self.cook_time}分钟, 状态是{self.cook_static}, 添加的调料有{self.condiments}'
digua1 = SweetPotato()
print(digua1)
digua1.cook(2)
digua1.add_condiments('酱油')
print(digua1)
digua1.cook(2)
digua1.add_condiments('辣椒⾯⼉')
print(digua1)
digua1.cook(2)
print(digua1)
digua1.cook(2)
print(digua1)
运行结果:
5.2 搬家具
5.2.1 需求
5.2.2 步骤分析
5.2.2.1 定义类
- 实例属性
-
房⼦地理位置
-
房⼦占地⾯积
-
房⼦剩余⾯积
-
房⼦内家具列表
- 实例⽅法
-
容纳家具
- 显示房屋信息
- 家具名称
- 家具占地⾯积
5.2.2.2 创建对象并调⽤相关⽅法
5.2.3 代码实现
5.2.3.1 定义类
class Furniture():
def __init__(self, name, area):
# 家具名字
self.name = name
# 家具占地⾯积
self.area = area
class Home():
def __init__(self, address, area):
# 地理位置
self.address = address
# 房屋⾯积
self.area = area
# 剩余⾯积
self.free_area = area
# 家具列表
self.furniture = []
def __str__(self):
return f'房⼦坐落于{self.address}, 占地⾯积{self.area}, 剩余⾯积{self.free_area}, 家具有{self.furniture}'
def add_furniture(self, item):
"""容纳家具"""
if self.free_area >= item.area:
self.furniture.append(item.name)
# 家具搬⼊后,房屋剩余⾯积 = 之前剩余⾯积 - 该家具⾯积
self.free_area -= item.area
else:
print('家具太⼤,剩余⾯积不⾜,⽆法容纳')
5.2.3.2 创建对象并调⽤实例属性和⽅法
bed = Furniture('双⼈床', 6)
jia1 = Home('北京', 1200)
print(jia1)
jia1.add_furniture(bed)
print(jia1)
sofa = Furniture('沙发', 10)
jia1.add_furniture(sofa)
print(jia1)
ball = Furniture('篮球场', 1500)
jia1.add_furniture(ball)
print(jia1)
六. 总结
- 创建类
class 类名():
代码
对象名 = 类名()
- 类外⾯
对象名.属性名 = 值
- 类⾥⾯
self.属性名 = 值
- 类外⾯
对象名.属性名
- 类⾥⾯
self.属性名 1
- __init__() : 初始化
- __str__() :输出对象信息
- __del__() :删除对象时调⽤