1. 调用关系图
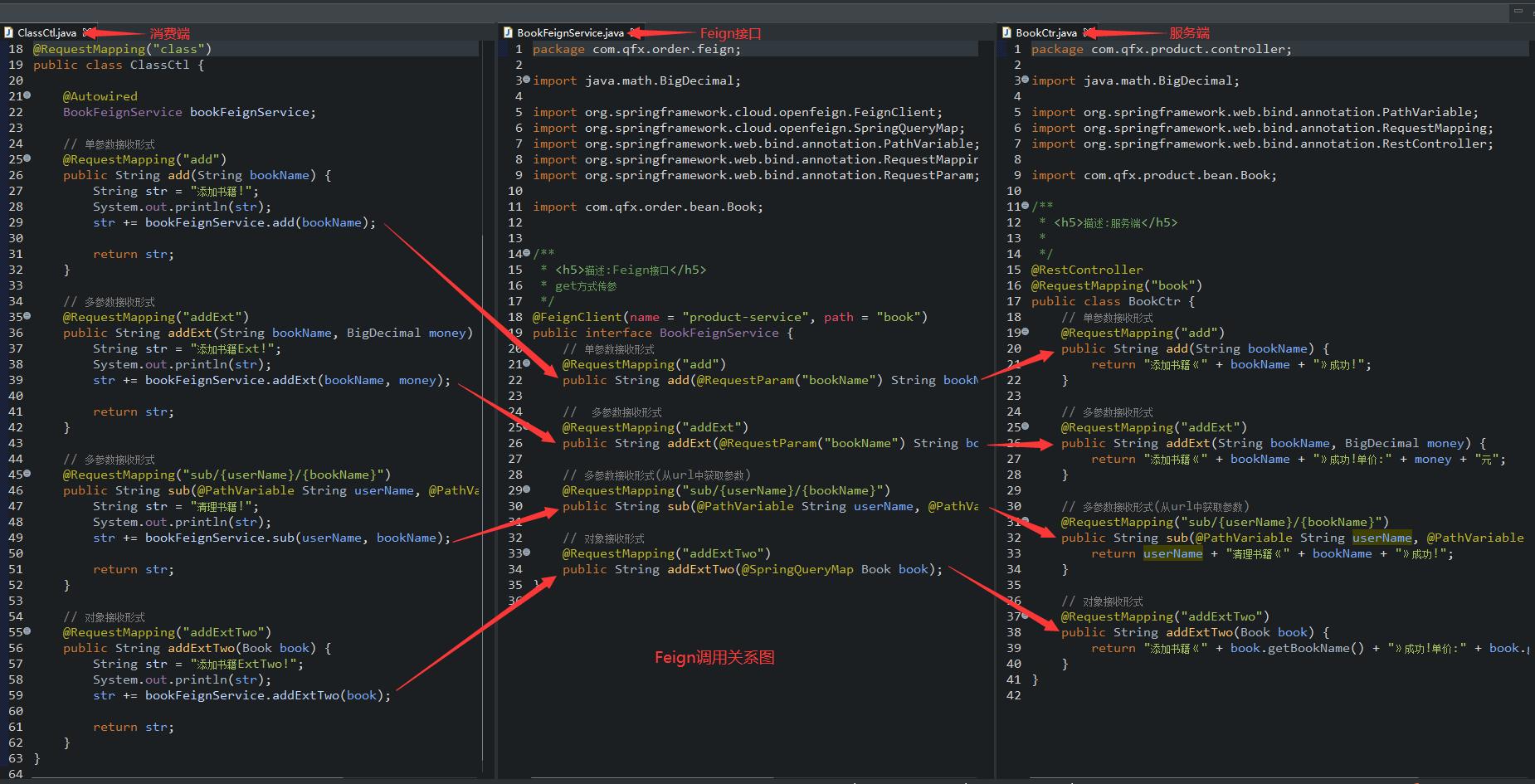
2. 实体类
import java.math.BigDecimal;
public class Book {
String bookName;
BigDecimal money;
public String getBookName() {
return bookName;
}
public void setBookName(String bookName) {
this.bookName = bookName;
}
public BigDecimal getMoney() {
return money;
}
public void setMoney(BigDecimal money) {
this.money = money;
}
}
3. 服务端
import java.math.BigDecimal;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.qfx.product.bean.Book;
/**
* <h5>描述:服务端</h5>
*
*/
@RestController
@RequestMapping("book")
public class BookCtr {
// 单参数接收形式
@RequestMapping("add")
public String add(String bookName) {
return "添加书籍《" + bookName + "》成功!";
}
// 多参数接收形式
@RequestMapping("addExt")
public String addExt(String bookName, BigDecimal money) {
return "添加书籍《" + bookName + "》成功!单价:" + money + "元";
}
// 多参数接收形式(从url中获取参数)
//sub方法中的参数userName和bookName会自动设置为URL中对应变量userName和bookName(同名赋值)的值,也可以自己指定,如:@PathVariable("userName") String userName
@RequestMapping("sub/{userName}/{bookName}")
public String sub(@PathVariable String userName, @PathVariable String bookName) {
return userName + "清理书籍《" + bookName + "》成功!";
}
// 对象接收形式
@RequestMapping("addExtTwo")
public String addExtTwo(Book book) {
return "添加书籍《" + book.getBookName() + "》成功!单价:" + book.getMoney() + "元";
}
}
4. Feign接口
import java.math.BigDecimal;
import org.springframework.cloud.openfeign.FeignClient;
import org.springframework.cloud.openfeign.SpringQueryMap;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import com.qfx.order.bean.Book;
/**
* <h5>描述:Feign接口</h5>
* get方式传参
*/
@FeignClient(name = "product-service", path = "book")
public interface BookFeignService {
// 单参数接收形式
@RequestMapping("add")
public String add(@RequestParam("bookName") String bookName);
// 多参数接收形式
@RequestMapping("addExt")
public String addExt(@RequestParam("bookName") String bookName, @RequestParam("money") BigDecimal money);
// 多参数接收形式(从url中获取参数)
//sub方法中的参数userName和bookName会自动设置为URL中对应变量userName和bookName(同名赋值)的值,也可以自己指定,如:@PathVariable("userName") String userName
@RequestMapping("sub/{userName}/{bookName}")
public String sub(@PathVariable String userName, @PathVariable String bookName);
// 对象接收形式
@RequestMapping("addExtTwo")
public String addExtTwo(@SpringQueryMap Book book);
}
5. 消费端
import java.math.BigDecimal;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.qfx.order.bean.Book;
import com.qfx.order.feign.BookFeignService;
/**
* <h5>描述:班级</h5>
*
*/
@RestController
@RequestMapping("class")
public class ClassCtl {
@Autowired
BookFeignService bookFeignService;
// 单参数接收形式
@RequestMapping("add")
public String add(String bookName) {
String str = "添加书籍!";
System.out.println(str);
str += bookFeignService.add(bookName);
return str;
}
// 多参数接收形式
@RequestMapping("addExt")
public String addExt(String bookName, BigDecimal money) {
String str = "添加书籍Ext!";
System.out.println(str);
str += bookFeignService.addExt(bookName, money);
return str;
}
// 多参数接收形式
//sub方法中的参数userName和bookName会自动设置为URL中对应变量userName和bookName(同名赋值)的值,也可以自己指定,如:@PathVariable("userName") String userName
@RequestMapping("sub/{userName}/{bookName}")
public String sub(@PathVariable String userName, @PathVariable String bookName) {
String str = "清理书籍!";
System.out.println(str);
str += bookFeignService.sub(userName, bookName);
return str;
}
// 对象接收形式
@RequestMapping("addExtTwo")
public String addExtTwo(Book book) {
String str = "添加书籍ExtTwo!";
System.out.println(str);
str += bookFeignService.addExtTwo(book);
return str;
}
}
6. 测试
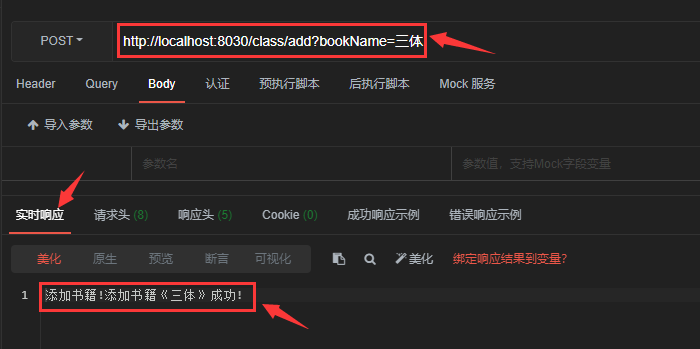
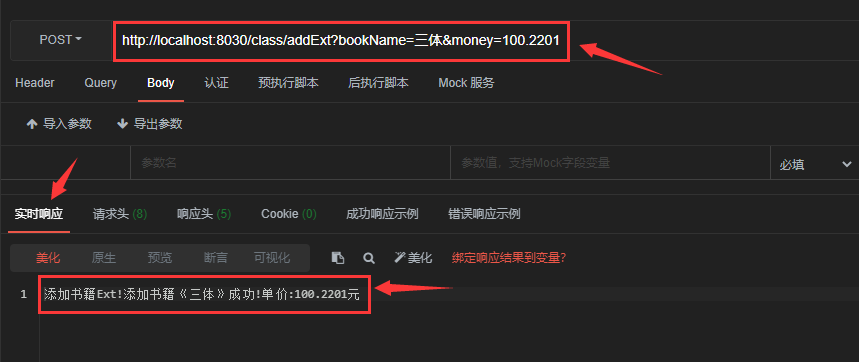
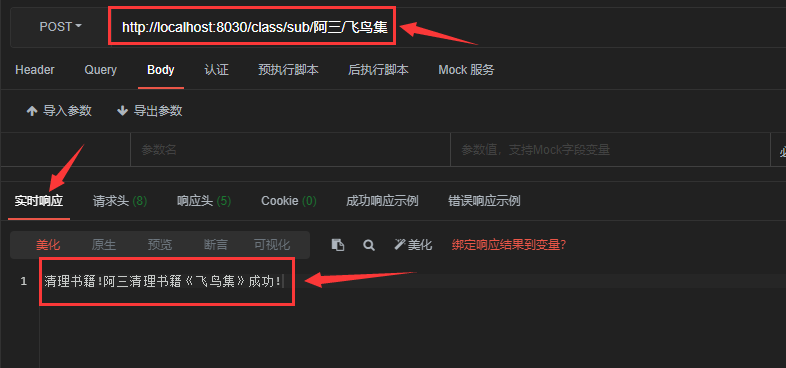
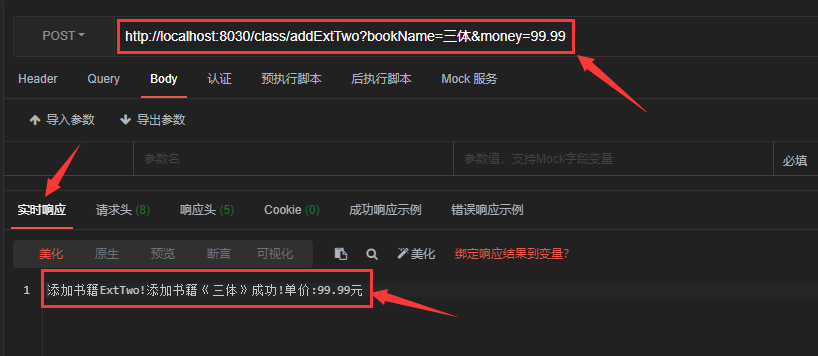